Simple Java program that performs arithmetic. The program is the solution to Deitel’s Java How to Program (9th Edition) Chapter 2 Exercise 2.15: Write an application that asks the user to enter two integers, obtains them from the user and prints their sum, product, difference and quotient (division).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
/** * *Website: www.cscprogrammingtutorials.com * * Exercise 2.15 - Arithmetic * This Program Calculates Simple Arithmetic of Addition, Difference (Subtraction), Product * (Multiplication) and Quotient (Division) of Any Two Numbers. * */ import java.util.Scanner; public class Arithmetic { public static void main (String [ ] args) { Scanner value = new Scanner (System.in); int num1; int num2; int add; int difference; int product; int division; System.out.println (); System.out.print ("Enter Your First Number: "); num1 = value.nextInt(); System.out.print ("Enter Your Second Number: "); num2 = value.nextInt(); add = num1 + num2; difference = num1 - num2; product = num1*num2; division = num1/num2; System.out.printf ("The Results Are: \nAddition = %d\nDifference = %d" + "\nProduct = %d\nQuotient = %d\n", add, difference, product, division); } } |
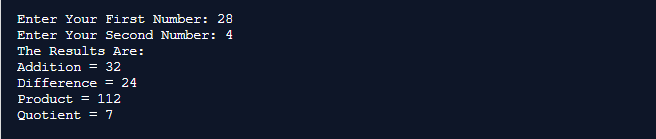