Java application to calculate the sum, average, product, smallest and largest of three numbers. The program below is the solution to Deitel’s Java How to Program (9th Edition) Chapter 2 Exercise 2.17.
Question: Write an application that inputs three integers from the user and displays the sum, average, product, smallest and largest of the numbers. Use the techniques shown in Fig. 2.15. [Note: The calculation of the average in this exercise should result in an integer representation of the average. So, if the sum of the values is 7, the average should be 2, not 2.3333….]
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 |
/** * * Exercise 2.17 - Arithmetic, Smallest And Largest * This Program Calculates And Displays The Sum, Average, Product, Smallest And Largest of * Three Integer Numbers. * */ import java.util.Scanner; public class Ex02_17 { public static void main (String [ ] args) { Scanner value = new Scanner (System.in); int num1; int num2; int num3; int sum; int average; int product; int smallest; int largest; System.out.print ("Enter Your First Number: "); num1 = value.nextInt (); System.out.print ("Enter Your Second Number: "); num2 = value.nextInt (); System.out.print ("Enter Your Third Number: "); num3 = value.nextInt (); sum = num1 + num2 + num3; average = (num1 + num2 + num3)/3; product = num1 * num2 * num3; smallest = num1; // assume smallest is the first number if (num2 < smallest) smallest = num2; if (num3 < smallest) smallest = num3; largest = num1; // assume largest is the first number if (num2 > largest) largest = num2; if (num3 > largest) largest = num3; System.out.printf ("\nSum = %d\nAverage = %d\nProduct = %d\nSmallest = %d\n" + "Largest = %d\n", sum, average, product, smallest, largest); } |
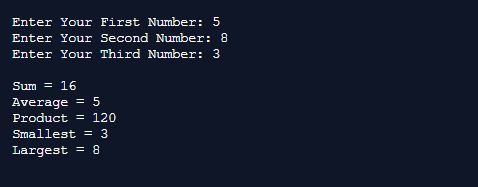
2 Comments
Thanks for pointing that out. I've corrected it.
Thanks for the answers, but you forgot to set int largest; in your answer