The program below separates the digits of a five-digit number using C programming language.
Question: Write a program that inputs one five-digit number, separates the number into its individual digits and prints the digits separated from one another by three spaces each. [Hint: Use combinations of integer division and the remainder operation.] For example, if the user types in 42139, the program should print 4 2 1 3 9
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 |
/* * Website: www.cscprogrammingtutorials.com * * Program to separate the digits of a five-digit number. * * Program Algorithm * ----------------- * declare variables to store user input(num) and each digits(dig1, dig2, dig3, dig4, dig5) * read the user input e.g. 42139 * assign the last digit of the number to dig5 ie 9 * divide the number by 10 ie 42139/10 = 4213 * calculate the remainder and assign the value to dig4 ie 3 * divide the number by 10 ie 4213/10 = 421 * calculate the remainder and assign the value to dig4 ie 1 * divide the number by 10 ie 421/10 = 42 * calculate the remainder and assign the value to dig4 ie 2 * divide the number by 10 ie 42/10 = 4 * calculate the remainder and assign the value to dig4 ie 4 * print the digits separated by three spaces ie 4 2 1 3 9 * */ #include <stdio.h> int main (void) { int num, dig1, dig2, dig3, dig4, dig5; printf("Enter a 5 digit whole number: "); scanf("%d", &num); dig5 = num % 10; num = num / 10; dig4 = num % 10; num = num / 10; dig3 = num % 10; num = num / 10; dig2 = num % 10; num = num / 10; dig1 = num % 10; printf("%d %d %d %d %d\n", dig1, dig2, dig3, dig4, dig5); return 0; } |
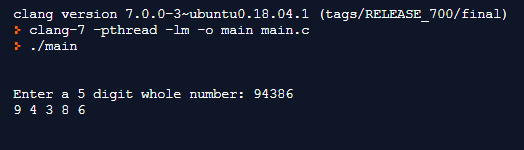
Click here to see other solutions to Deitel C How To Program exercises. Here is the same program to separate digits of a number written in Java using another algorithm.
1 Comment
This article is awesome for all beginner level learner. thanks for this blog.